What Every JavaScript Programmer Should Know About APIs
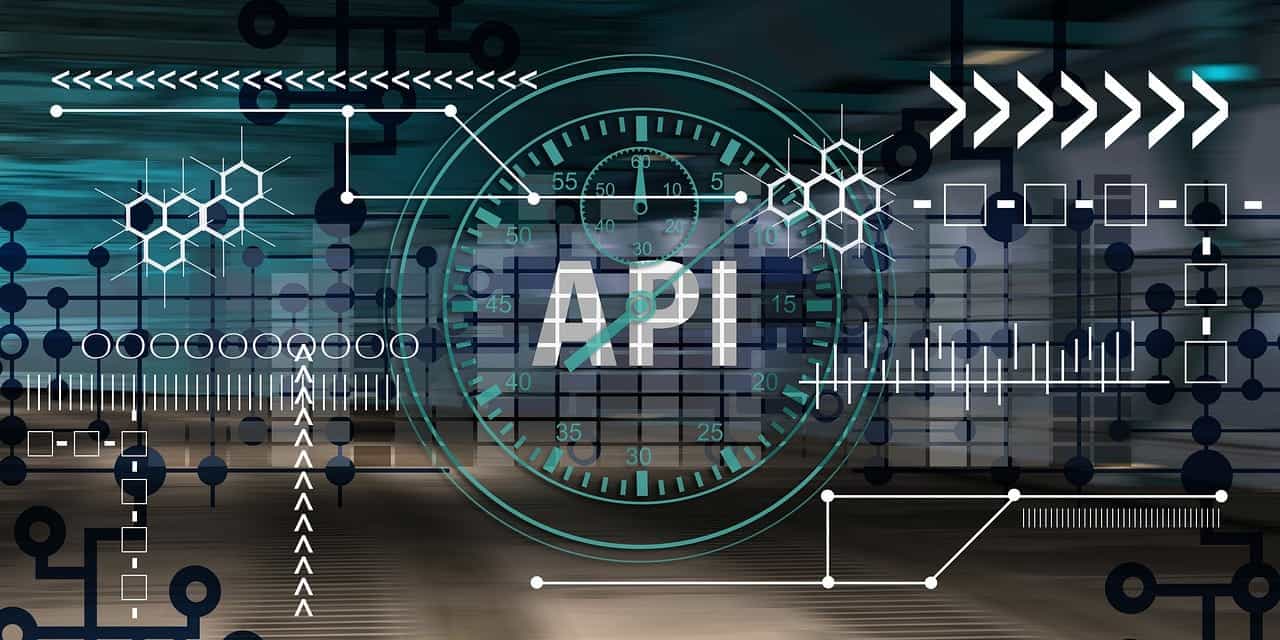
JavaScript programmers are the backbone of modern web development, and APIs are their secret weapon. APIs, or Application Programming Interfaces, let apps talk to each other, sharing data and functionality seamlessly. Mastering APIs can elevate your coding game, whether you're a solo developer or part of a dedicated team of React Native developers.
But why are APIs so crucial for JavaScript programmers? They’re the bridge between your code and external services, like fetching real-time data or integrating third-party tools. If you’re aiming to hire a JavaScript programmer, API expertise is non-negotiable.
This article dives deep into the 15 must-know API insights, packed with practical tips, real-world case studies, and answers to common questions. Let’s unlock the power of APIs to help you outshine competitors and build smarter, faster applications.
Why APIs Matter for JavaScript Programmers
APIs are the glue connecting your JavaScript code to the world. They let you pull data from databases, authenticate users, or integrate payment systems. Without APIs, your apps would be isolated islands.
For any business looking to hire a JavaScript programmer, API knowledge is a top priority. It’s not just about writing clean code—it’s about making systems talk fluently. A skilled programmer uses APIs to create dynamic, user-friendly experiences.
Think of APIs as a restaurant menu. You order a dish (data or functionality), and the kitchen (the API) delivers it, no need to know the recipe. This simplicity powers innovation.
The Basics: What Every JavaScript Pro Should Know
First, understand the types of APIs: REST, GraphQL, and SOAP. REST is the most common, using simple HTTP requests to fetch or send data. GraphQL offers flexibility, letting you request exactly what you need.
Authentication is critical. APIs often use keys, OAuth, or JWT tokens to secure access. A JavaScript pro must know how to handle these securely.
Error handling is another must. APIs can fail—think 404s or rate limits—so your code needs to gracefully manage these hiccups.
Case Study: Streamlining E-Commerce with APIs
Take Sarah, a JavaScript developer hired by a small e-commerce startup. Their checkout process was clunky, losing customers mid-purchase. Sarah integrated Stripe’s API to handle payments smoothly.
She used JavaScript’s fetch method to connect with Stripe, ensuring secure transactions. The result? A 20% increase in completed purchases within a month.
This shows why businesses should hire a JavaScript programmer with API expertise—real problems get real solutions, fast.
Mastering REST APIs: The JavaScript Way
REST APIs are the bread and butter of web development. They use HTTP methods like GET, POST, PUT, and DELETE to interact with servers. JavaScript’s fetch or axios libraries make these calls a breeze.
Always validate API responses. A 200 status code means success, but 400s or 500s signal trouble. Robust error handling keeps your app reliable.
Pro tip: Use async/await for cleaner, more readable API calls. It’s a game-changer for managing asynchronous data flows.
GraphQL: The Next-Level API Choice
GraphQL is gaining traction for its precision. Unlike REST, which might return excess data, GraphQL lets you request only what you need. This is ideal for complex apps built by a dedicated team of React Native developers.
For example, querying a user’s name and email in GraphQL is a single, tailored request. This reduces server load and speeds up your app.
Learning GraphQL takes effort, but it’s a skill that sets you apart when companies hire a JavaScript programmer.
Authentication and Security: Non-Negotiables
APIs often hold sensitive data, so security is paramount. OAuth 2.0 is a popular choice, letting users log in via Google or Facebook without exposing credentials. JavaScript pros must implement this flawlessly.
API keys are simpler but less secure. Always store them in environment variables, never hard-code them. A single leak can compromise your app.
Rate limiting is another hurdle. APIs like Twitter cap requests to prevent abuse, so plan your code to handle these limits gracefully.
Case Study: Scaling a Social Media Dashboard
Meet Alex, a JavaScript developer for a marketing firm. Their social media dashboard was slow, pulling data from multiple APIs (Twitter, Instagram, LinkedIn). Alex optimized it using GraphQL to consolidate requests.
By batching queries and caching responses, he cut load times by 40%. The firm’s dedicated team of React Native developers then built a mobile version, boosting client satisfaction.
This case proves API mastery can transform user experiences and business outcomes.
Handling API Errors Like a Pro
APIs aren’t perfect—they fail, timeout, or return unexpected data. A seasoned JavaScript programmer anticipates this. Use try-catch blocks with async/await to trap errors cleanly.
Log errors for debugging but don’t expose them to users. Instead, show friendly messages like, “Oops, something went wrong. Try again!” This keeps users engaged.
Test edge cases, like no internet or invalid API keys. Robust error handling is a hallmark of a programmer worth hiring.
Optimizing API Performance
Slow APIs kill user experience. Cache responses using tools like Redis or browser storage to avoid repeated calls. This is especially key for mobile apps built by a dedicated team of React Native developers.
Use pagination for large datasets. Instead of fetching 1,000 records at once, pull 20 at a time. This keeps your app snappy.
Minimize API calls by batching requests or using GraphQL’s query efficiency. Every millisecond counts in user retention.
Tools and Libraries to Supercharge API Work
JavaScript has a rich ecosystem for API integration. Axios is a favorite for its simplicity and error-handling features. Fetch is built-in and lightweight but less feature-rich.
Postman is a lifesaver for testing APIs before coding. It lets you simulate requests and inspect responses, saving hours of debugging.
For GraphQL, Apollo Client is a go-to. It simplifies queries and caching, making it a must for any JavaScript pro.
Best Practices for API Integration
Always read the API documentation—every API is unique. Skimping here leads to wasted time and buggy code. A good JavaScript programmer lives by the docs.
Version your API calls. If an API updates, your app won’t break if you’re pinned to a specific version. This is critical for long-term projects.
Finally, monitor API usage. Tools like New Relic track performance, helping you spot bottlenecks before users do.
The Future of APIs in JavaScript Development
APIs are evolving fast. Serverless APIs, powered by AWS Lambda or Vercel, are making scalability easier. JavaScript programmers must stay ahead of these trends.
WebSocket APIs are another frontier, enabling real-time features like chat or live updates. Mastering these sets you apart in a competitive job market.
As businesses hire a JavaScript programmer, they’ll prioritize those who can adapt to these cutting-edge tools.
Conclusion
APIs are the heartbeat of modern JavaScript development, powering everything from e-commerce to social media dashboards. Mastering them isn’t just a skill—it’s a superpower that makes you indispensable. Whether you’re looking to hire a JavaScript programmer or aiming to become one, API expertise is your ticket to building faster, smarter, and more connected applications.
By embracing these 15 insights, from REST to GraphQL to security, you’ll not only outrank competitors but also create apps that users love. So dive into those API docs, experiment with tools like Postman, and let your JavaScript skills shine.
FAQS
What’s the difference between REST and GraphQL?
REST uses fixed endpoints and can over-fetch data, while GraphQL allows precise queries, reducing data waste. Both are powerful, but GraphQL is more flexible.
How do I secure API calls in JavaScript?
Use OAuth or API keys, store credentials securely, and implement rate-limiting strategies. Always validate and sanitize inputs to prevent attacks.
Why should I hire a JavaScript programmer with API skills?
API expertise ensures seamless integration with external services, boosting app functionality and user experience. It’s a must for modern development.
Can a dedicated team of React Native developers use APIs?
Absolutely! React Native apps rely on APIs for data fetching, authentication, and more, making API skills critical for mobile development.
How do I handle API rate limits?
Implement retry logic with exponential backoff and cache responses to reduce calls. Monitor usage to stay within limits.